

  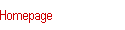
  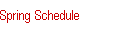
  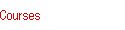
  
  
  
  
  
  
  
  
  
  
  
  
  
  
  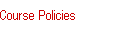
  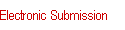
  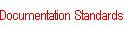
  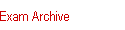
  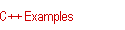
  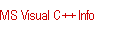
  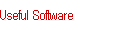
  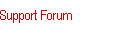
  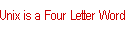
  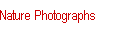

![[Home]](../icons/up.png)
![[Rich]](../icons/prev.png) ![[Home]](../icons/home.png) ![[Rich]](../icons/next.png)
![[Author]](../icons/author.png) |
CS182 -- Detailed Objectives
At the time of Exam I, a student should be able to:
- Describe and identify the main components found in the typical
computer architecture.
- Describe and identify the steps used to develop software.
- Understand the basic tenet of the object-oriented model (i.e.,
the coupling of attributes and methods).
- Suggest possible attributes and methods of simple everyday
objects.
- Apply the rules for identifier naming used in C++ at a level
sufficient to suggest valid names and to identify invalid names.
- Declare and initialize simple data objects of the following
types:
- short int
- int
- long int
- char
- float
- double
- bool
- Apply the rules for constants of the types listed above.
- Understand and apply the concept of just-in-time declaration.
- Identify the lifetime of data objects from a code listing.
- Write and analyze expressions involving the following operators:
=,+,-,*,/,%,++,--,+=,-=,/=,*=,%=
- Use the cin and cout
objects to perform simple I/O.
- Describe and apply the difference between the pre and post
forms of the ++ and --
operators.
- Write and analyze boolean expressions involving: ==,<,>,>=,<=,!=.&&,||,!
- Describe and apply the precedence and association rules for
any operator listed in the objectives above.
- Describe the effects that the keyword static
has on object in terms of lifetime and behavior.
- Write and analyze short sections of C++ that use strings
for the following operations:
- Declaration and initialization
- I/O
- Concatenation
- Write and interpret C++ code involving selection statements
of the following types:
- if
- if else
- if else if ...
- switch ... case
- ?: (conditional operator)
- Write and interpret C++ code involving loop statements of
the following types:
- Understand and describe the role of the code block
{} in C++.
- Describe the usage of the break
statement in a switch...case
and looping statements.
- Describe the effects that the modifier unsigned
has on integer data types.
- Write short C++ programs including the use of #include
and using namespace std;
- Analyze short C++ programs and describe what they do.
At the time of Exam II, a student should be able to:
- Meet all of the above education objectives.
- Implement simple C++ programs using Microsoft Visual Studio .NET.
- Write simple use cases.
- Understand the implicit type conversions of C++ and be able
to use static_cast to perform
an explicit type conversion.
- Describe the difference between the declaration (prototype)
and definition of a function.
- Understand and use the C++ parameter passing mechanism for
functions.
- Use the #define preprocessor
directive in its simplest form.
- Explain the difference between ""
and <> with the #include
directive.
- Describe the differences between endl,
flush, and '\n' as they
apply to cout.
- Describe the role and purpose of cerr
and clog.
- Use simple stream manipulators like dec,
hex, oct, setw, fixed, scientific, setprecision, setfill, left,
right, noboolalpha, and boolalpha.
- Explain the similarities between iostream and file streams.
- Use file streams to open files, read from them, and write
to them.
- Know how to test a file stream for a failed operation or
to detect when the end of a file has been reached.
- Use the basic functions in the cmath
library.
- Use the assert function/macro
to do simple error checking.
- Write and interpret simple C++ functions including proper
documentation.
- Demonstrate proper use of the return
statement.
- Describe the difference between the scope and lifetime of
objects and functions.
- Identify the scope of objects and functions in a program.
- Describe a name conflict and C++'s way of dealing with it.
- Describe the difference between value and reference parameters
and the syntax for each.
- Know when to use reference and const reference parameters.
At the time of the Final Exam, a student should be able to:
- Meet all of the above educational objectives.
- Describe and apply the variations in functions due to default
parameters and overloading.
- Identify the signature of a function.
- Describe the organizational differences between console mode
and graphical user interface (GUI) programs.
- Describe the differences, advantages, and disadvantages of
the following container objects (including their storage mechanisms):
- arrays
- strings
- vectors
- lists
- Declare and index arrays of up to two dimensions.
- Describe the differences between char arrays, c-style strings,
and ANSI string objects.
- Describe, understand, and perform basic operations using
vectors:
- creating
- indexing
- push_back
- resize
- clear
- size
- empty
- Describe and understand, and perform basic operations using
strings:
- creating
- indexing
- concatenating
- substrings
- comparison
- insertion
- replacement
- erasing
- substring search
- I/O
- Describe and use stringstreams to format and parse character
data.
- Describe and apply the syntax of iterators to simple applications.
Acknowledgement
This page was based on a similar set of pages developed originally by
Dr. Henry Welch.
|