There is a more recent offering of this course. This is an archive of the
course which was offered in Winter 2018-2019.
CS1021
Homework
Homework
Opportunities to practice
## Week 1 (Review)
* [CodingBat: shiftLeft](http://www.codingbat.com/prob/p105031)
* [CodingBat: tripleUp](http://www.codingbat.com/prob/p137874)
* [CodingBat: seriesUp](http://www.codingbat.com/prob/p104090)
* [CodingBat: copyEvens](http://www.codingbat.com/prob/p134174)
* [CodingBat: fix34](http://www.codingbat.com/prob/p159339)
* Exercise: 10.7, 10.9, 10.10
* [CodingBat: lucky13](http://www.codingbat.com/prob/p194525)
* [CodingBat: sum28](http://www.codingbat.com/prob/p199612)
* [CodingBat: centeredAverage](http://www.codingbat.com/prob/p136585)
* The above centeredAverage problem with an ArrayList instead. (`public int centeredAverage(ArrayList nums)`)
* [CodingBat: linearIn](http://www.codingbat.com/prob/p134022)
* Exercise: 10.10, 12.2, 12.4, 12.9, and 12.13
## Week 2
* Exercise: 13.1, 13.2, 13.3, 13.6, 13.10, and 13.17
## Week 3
* Exercise: 14.1, 14.3, 14.6, 14.7, 14.10, 14.11, and 14.13
## Week 4
1. Write a simple calculator program that looks like this:
[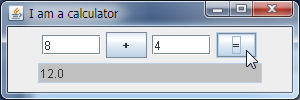](hw4.png)
The program display the result of adding the two numbers together.
1. Modify the program so that the user cannot modify the answer text field.
1. Modify the program to color the background of the result text field red if the result is negative, otherwise the background should be light gray.
1. Modify the program so that if the + is clicked, it turns into a - button; if clicked again, it turns into a * button; if clicked again, it turns into a / button; and if clicked again, returns to a + button.
1. Modify the program so that if = button is clicked, the appropriate math operation is used to produce the result shown in the last text field.
1. Modify the program so that hitting enter while in either of the first two text fields is equivalent to pressing the = button.
1. Suppose you were required to use at least one anonymous inner class in your implementation. Where would you use it, and why?
1. Modify your program so that it displays "ERROR" with a red background in the result field if either of the input textfields contain invalid information.
1. Modify your program to add % as a fifth operator option.
## Week 5
What follows are a number of GUI layouts written in Swing. For each image, create a JavaFX application that looks similar. You may need to learn and use UI controls that we have not discussed in class.
1. [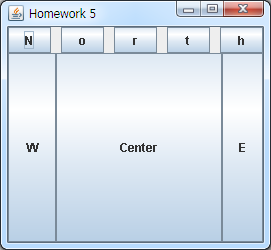](hw5a.png)
1. [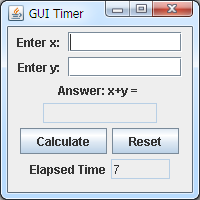](hw5b.png)
1. [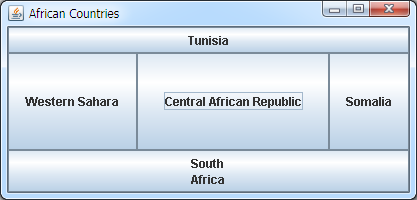](hw5c.png)
1. [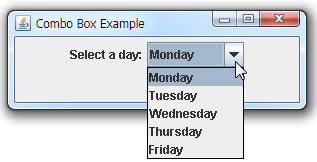](hw5d.png)
1. [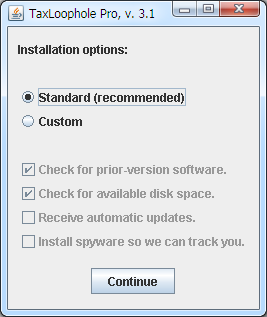](hw5e.png)
1. [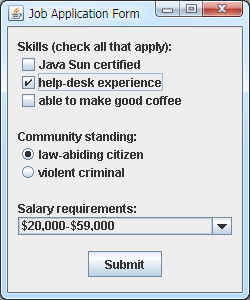](hw5f.png)
1. [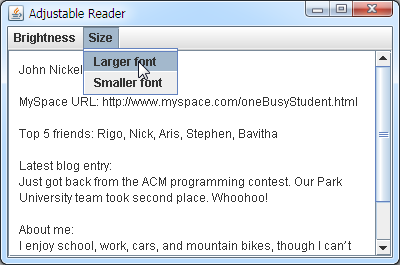](hw5g.png)
1. Make the program shown in **2** functional.
1. Add functionality to the program shown in **5** such that the checkboxes become active when the **Custom** radio button is selected.
1. For the program in **6** display a dialog box stating "Thanks for your application. We'll get back to you." or "Thanks for your application. Unfortunately we don't have any jobs available at this time." depending on which community standing radiobutton has been selected.
1. Make the program shown in **7** functional. The Brightness menu has two options that change the background color of the text area.
## Week 6
1. Explain how exception handling increases the robustness of software
1. Explain why there are multiple classes that derive from the `Exception` class
1. What happens when an exception is thrown during the execution of a program but never caught
1. Consider the following stack trace:
```
java.lang.ArithmeticException: / by zero
at wk6.Homework.method4(Homework.java:26)
at wk6.Homework.method3(Homework.java:22)
at wk6.Homework.method2(Homework.java:18)
at wk6.Homework.method1(Homework.java:11)
at wk6.Homework.main(Homework.java:6)
```
What caused the exeception and where (which method) did the exception occur?
1. Suppose that `method1()` (from the previous problem) is implemented as follows:
```
private static void method1(String string) {
try {
method2(string + " birthday");
} catch (ArithmeticException e) {
e.printStackTrace();
} catch (Exception e) {
System.out.println(e.getMessage());
}
System.out.println("In method1");
}
```
What will be the output of the program?
1. What will happen if the method is implemented as follows:
```
private static void method1(String string) {
try {
method2(string + " birthday");
} catch (Exception e) {
e.printStackTrace();
} catch (ArithmeticException e) {
System.out.println(e.getMessage());
}
System.out.println("In method1");
}
```
1. Describe when throwing an exception is preferred over including error handling code at the source of the error.
## Week 7
For these homework problems, you will be using this [Complex.java](Complex.java) class.
1. Implement a `write(DataOutputStream out)` method in the `Complex` class that will write the fields of the `Complex` object to a binary output stream.
1. Implement a `read(DataInputStream in)` method in the `Complex` class that will read a complex number from a binary input stream and return a `Complex` object with the value read from the stream.
1. Write a driver program that creates four complex numbers: 1.0 + 0.0i, -2.3 - 5.0i, 0.0 + 3.0i, and 8.0 + 8.0i and writes them to a binary file.
1. Write another driver program that reads two complex numbers from a binary file and displays them to the console in both cartesian and polar form.
1. Implement a `write(PrintWriter out)` method in the `Complex` class that will write the fields of the `Complex` object to a text output stream.
1. Implement a `read(Scanner in)` method in the `Complex` class that will read a complex number from a text input stream and return a `Complex` object with the value read from the stream.
1. Write another driver program that creates four complex numbers: 1.0 + 0.0i, -2.3 - 5.0i, 0.0 + 3.0i, and 8.0 + 8.0i and writes them to a text file. All numbers should be on one line and separated by commas.
1. Write another driver program that reads all complex numbers in a text file (assume no other data is in the file and that the format described in the previous activity is used) and displays them to the console in cartesian form.
1. Modify the `Complex` class so that it can be written to an `ObjectOutputStream`, and write a driver program that creates four complex numbers: 1.0 + 0.0i, -2.3 - 5.0i, 0.0 + 3.0i, and 8.0 + 8.0i and writes them to a binary file as objects.
1. Write another driver program that reads four complex numbers that have been written to a binary file as objects, and displays them to the console in polar form.
## Week 8
For these homework problems, you will be using this [Complex.java](Complex.java) class.
1. Implement a method, `getReal()`, that accepts a `List` and returns a list of all the complex numbers where the imaginary component is zero.
1. Write a class, `IsReal`, that implements the [`Predicate`](http://javadoc.taylorial.com/java.base/util/function/Predicate.html) interface. The `test()` method must return `true` if and only if the imaginary component of the complex number is zero.
1. Write a method, `getSpecified()`, that accepts a `List` and a `Predicate` and returns a list of all the complex numbers for which the predicate is true.
1. Write a code snippet that calls `getSpecified()` using a lambda expression that produces true whenever the magnitude of the complex number is greater than 27 for the predicate.
1. Make use of a [`Stream`](http://javadoc.taylorial.com/java.base/util/stream/Stream.html) to generate the same list as required by the previous problem. You may not use `getSpecified()`. Instead you should use `Stream.filter()` and `Stream.collect()`.
1. Use the `List.forEach()` method to display each complex number on a separate line.
1. Make use of a `Stream` and `DoubleStream` to calculate the average angle, in degrees, of the complex numbers in a list.
1. CodingBat: [copies3](http://codingbat.com/prob/p181634)
1. CodingBat: [lower](http://codingbat.com/prob/p186894)
1. CodingBat: [noLong](http://codingbat.com/prob/p194496)
1. CodingBat: [two2](http://codingbat.com/prob/p148198)
1. CodingBat: [noTeen](http://codingbat.com/prob/p137274)
1. CodingBat: [square56](http://codingbat.com/prob/p132748)