

  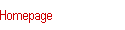
  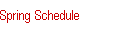
  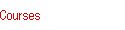
  
  
  
  
  
  
  
  
  
  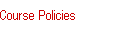
  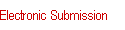
  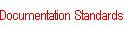
  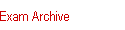
  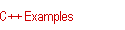
  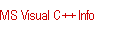
  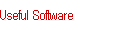
  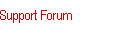
  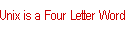
  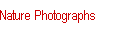

![[Home]](../icons/up.png)
![[Rich]](../icons/prev.png) ![[Home]](../icons/home.png) ![[Rich]](../icons/next.png)
![[Author]](../icons/author.png) |
CS183 -- Detailed Objectives
At the time of the midterm exam, a student should be able to:
- Describe the the software lifecycle and explain its iterative nature.
- Describe the linked list storage configuration.
- Compare and contrast the vector and list as container types.
- Describe the purpose and use of iterators including the motivation for
the four basic types (iterator,
const_iterator,
reverse_iterator, and
const_reverse_iterator).
- Write and interpret code using lists and iterators.
- Describe the purpose and use of a reference.
- Describe the purpose and use of a pointer.
- Compare and contrast references vs. pointers.
- Write and interpret code using pointers to access and manipulate
objects.
- Describe the purpose and apply the NULL
pointer where appropriate.
- Describe the similarities and differences between pointers and
arrays.
- Describe the basic properties of an abstract data type.
- Explain the motivation for using abstract data types
including the advantages of doing so.
- Write and interpret the basic syntax for C++ classes.
- Develop the prototypes and write C++ member functions for the
following:
- Constructors
- Copy Constructors
- Assignment/operator=
- Destructors
- Accessors
- Mutators
- Facilitators
- Supporting operators
- Arithmetic
- Relational (e.g. operator==)
- Stream (e.g. << and >>)
- Explain the purpose and use of the this pointer.
- Explain the effect the designation const,
static, and friend
have on functions.
- Explain the C++ automatic type conversion mechanism and describe how
to best take advantage of it.
- Interpret and draw UML class diagrams and the syntax they use to
describe the relationships between classes.
- Use strategies like use cases and classification to determine
appropriate classes, methods, and attributes as part of the design
of a software solution to a problem.
At the time of the Final Exam, a student should be able to:
- Explain the similarities and differences between static, automatic
and dynamic objects and the locations in memory where they are
stored.
- Write and interpret code that uses new,
delete, and delete []
to manipulate dynamic objects.
- Describe a memory leak and explain why it is undesirable.
- Understand the organization of multi-source file C++ code.
- Describe which code should go in .h and
.cpp files.
- Describe the difference between declarations and definitions.
- Demonstrate proper use of the #include
and #ifndef compiler directives.
- Describe the difference between the composition and inheritance
mechanisms.
- Describe the following inheritance issues:
- Base class
- Derived class
- Early (static) versus late (dynamic) binding
- Upcasting
- Overriding
- The keyword virtual
- Pure virtual
- Abstract base class
- Interpret and write C++ code using inheritance.
- Describe the differences between private,
protected, and public
access specifications.
- Describe what a friend is.
- Define the term polymorphism and be able to describe the C++ language
mechanisms which implement it.
- Interpret and write C++ code for functions and classes which
use templates.
- Interpret and write container classes (e.g. vector and list)
using templates.
- Interpret and write code which use and implements iterators.
- Including the syntax for overriding
operator++ and
operator--.
- Describe the C++ namespace mechanism and know how to build
namespaces.
- Describe the purpose and use of the C++ exception handling mechanism.
- Interpret and write simple code using the C++ exception handling
syntax involving throw,
try, and catch.
- Describe and apply common STL algorithms such as
find, sort, etc.
Acknowledgement
This page was based on a similar set of pages developed originally by
Dr. Henry Welch.
|