Objectives
- Translate UML class diagrams into source code
- Construct software which using an abstract class and polymorphism
- Employ aggregation and composition in the development of software
- Use javadoc to determine appropriate use of an unfamiliar class
Assignment
Shape Implementations
Your assignment is to write the Shape
,
Point
,
Triangle
,
LabeledTriangle
,
Rectangle
,
LabeledRectangle
,
and Circle
classes.
The classes are part of an inheritance hierarchy that is shown
in the UML class diagram below. Note that the
Shape
class is an abstract
class. Also, be sure that your implementation adheres to
the visibility modifiers shown in the diagram.
Place each of these classes in a package that uses your MSOE username as the name.
Implementation specific details for these classes is found in the javadoc for these classes.
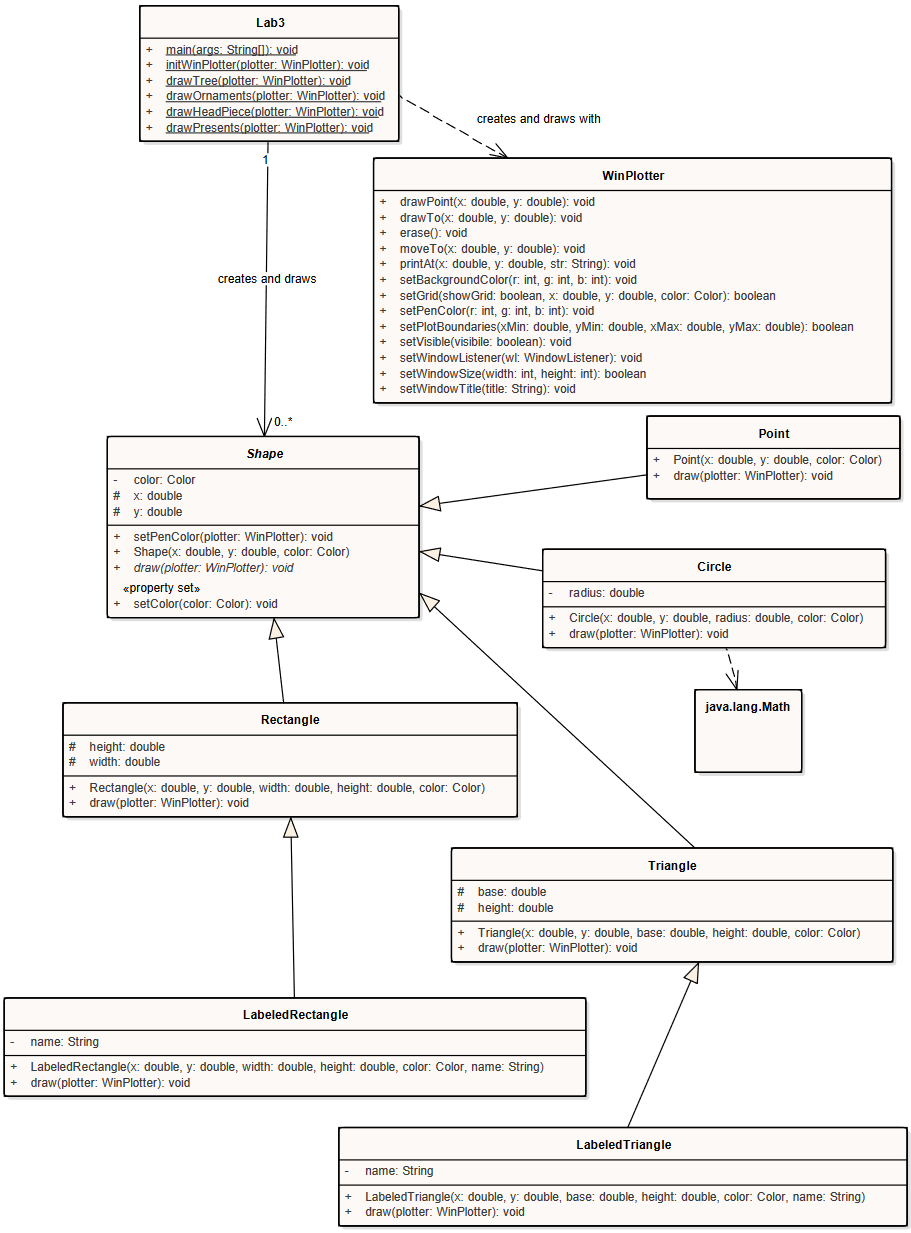
Please note that the class named Lab3
in the above diagram
should be named ShapeCreatorApp
.
ShapeCreatorApp
The ShapeCreatorApp class has
already been implemented. You will need to add the correct
import
statement(s) for your
shape classes. No other changes need to be made to the
ShapeCreatorApp
class.
The program makes use of the classes that you must implement to create the following:
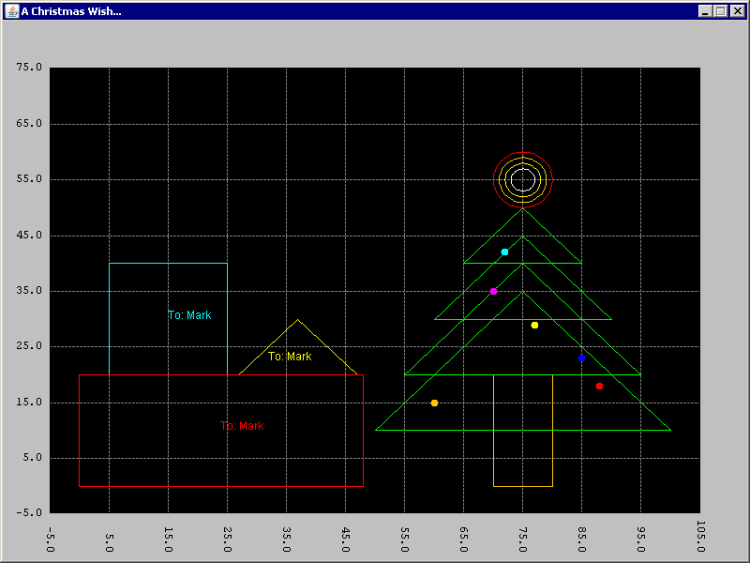
WinPlotter
The program relies on the WinPlotter
class. Dr. Hornick wrote the WinPlotter
class,
and the documentation for the class may be found
here.
You will need to download and then add the
WinPlotter.jar
file to your build
path (see instructions below).
Accessing the .jar File
For this assignment, you will need to make use of the WinPlotter.jar.
In order to be able to access the WinPlotter
class in the
.jar
file:
- Create a libs folder in your IntelliJ project folder
- Download the WinPlotter.jar file and save it in the newly created libs folder.
- Select File -> Project Structure
- Select Modules from the Project Settings on the left
- Select the Dependencies tab on the right
- Select the + on the far right
- Select Libraries -> Java from the drop-down menu
- Select the libs folder you created above and click OK
- In the Configure Library dialog box that appears, accept the default settings: Name = libs and Level = Project Library
- Click OK to close the Project Structure window
You should now have access to the
edu.msoe.se1010.winPlotter.WinPlotter
class in your project
code.
Details
- Every class in this inheritance hierarchy, except the
Shape
class implements thedraw
method. - The
draw
methods all take a single argument, which is a reference to theWinPlotter
object created at the beginning of themain
method in theShapeCreatorApp
class. As a result, all shapes use the sameWinPlotter
object for drawing. - Each implementation of the
draw
method must use methods from theWinPlotter
class to draw the desired shape. - To help you understand what you must write for each class (and how to draw each shape), read the Javadoc for the Shape-derived classes.
Just For Fun
Feel free to create your own version of the ShapeCreatorApp class that draws a picture of your own choosing. You can submit a screen shot of this as the supplemental file on the upload page.
Lab Deliverables
All other students should refer Blackboard
Acknowledgment
This laboratory assignment was developed by Dr. Mark Hornick and Dr. Chris Taylor.