Overview
You will be building a simple service that functions as Domain Name System (DNS). A DNS is a translator between a domain name and the corresponding IP (Internet Protocol) address. For example, if the domain name "www.msoe.edu" is supplied to the system, the IP address "155.92.194.118" would be returned. A second function should be the ability to add a new IP address for a domain name (i.e. create a new domain/IP mapping), update a mapping or remove a mapping.
Assignment
You will need to create the following classes:
Simulator
- Contains the GUI andmain
methodDomainName
- Represents a domain nameIPAddress
- Represents an IP addressDNS
- The Domain Name System
The user will interact with your program via a GUI similar to the mockup below:
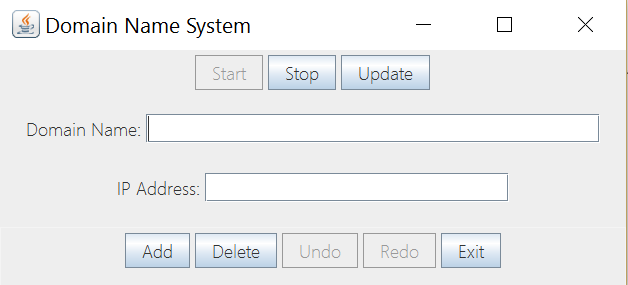
When the program starts, only the Start and Exit buttons should be enabled. Once started, the other buttons should be enabled and the Start button should be disabled. The text fields should only be enabled when the Start button is disabled. If the user hits enter while in the domain name entry text field, the program should look up the IP address for the domain and display it in the IP Address field (or display "NOT FOUND" if the domain name was not found on the server. Pressing enter in the IP Address text field should not do anything.
Domain Names
The user interface allows the user to lookup a name using a single text line dialogue box that can take up to 253 characters. The format of the text should be that the string must start with an alphanumeric character followed by a sequence of legal characters. In addition to alphanumeric characters, periods . and dashes - are considered to be a legal characters (just not at the beginning of the domain name). Additionally, two periods must not be placed in series. The following are all legal names:
- google.com
- localhost
- ww4.google.com
- t-a-y-l-o-r.com
- msoe.edu
Domain names are case insensitive.
At a minimum, your DomainName
class must have the following interface:
DomainName(String domain)
- Creates a new DomainName object. If the domain passed to the constructor is invalid, aIllegalArgumentException
must be thrown.String toString()
- Returns a string representing the DomainName.boolean equals(Object domain)
- Returns true if and only ifdomain
is equal to this object (recall domain names are case insensitive).
IP Addresses
A valid IP 4 address must be of the form of four segments separated by periods: [0-255].[0-255].[0-255].[0-255] with no other characters permitted. The following are legal IP addresses:
- 145.0.0.5
- 192.168.0.0
- 127.0.0.0
- 254.106.106.106
The following are illegal addresses:
- 14a.0.0.5
- a.b.c.d
- 301.456.789.124
At a minimum, your IPAddress
class must have the following interface:
IPAddress(String newAddress)
- Creates a new IPAddress object. If the IPAddress passed to the constructor is invalid, aIllegalArgumentException
must be thrown.String toString()
- Returns a string representing the IPAddress.boolean equals(Object address)
- Returns true if and only ifaddress
has the same four address subfields.
The DNS
The domain name system should use a class that implements the Map
interface as an attribute when implementing the domain name system. The DNS should be a class and have the following interface:
DNS(String filename)
- Constructor that sets the filename to be used to store the DNS entries.boolean start()
- Starts the DNS by reading in the names and initializing the map (see Initialization below). Returns true if it was already started or if it was started successfully.boolean stop()
- Terminates the server. However, it can be restarted (so don't exit the whole program). The DNS table should be written to the DNS file (specified in the constructor). Returns true if it was already stopped or if it was stopped successfully.IPAddress lookup(DomainName domain)
- Returns the IP address for the specified domain. If the domain name is not found, it returnsnull
. Note: if the server is currently not started, this method should returnnull
regardless of what is passed to it.IPAddress update(String command)
- See Update description below.
When reading from a file, if a line contains an invalid domain name or IP address, an error message must be sent to System.err
and the line in the file is ignored. When interactive with the GUI, if an invalid domain name or IP address is entered, an error dialog must be displayed indicating the error and the command should not be processed.
Initialization
When start()
is called, your DNS object must initialize and populate the map by reading in the file. Here is a sample file to use: dnsentries.txt. As we noted before, we can have multiple domain names map to single IP address, so it is alright if we have duplicate domain name to IP address mappings, just not the other way around.
Update
Note: if the server is not started, this method should just return null
.
We can receive a series of updates to the DNS. We will use a single file called updates.txt that contains multiple lines, each with a command (ADD or DEL), IP address, and domain name that is used to update our map. Each line of the file must be processed with a separate call to update()
. There are two types of updates:
ADD IPAddress DomainName DEL IPAddress DomainName
If the command is not ADD or DEL, the domain name is invalid, or the IPAddress is invalid, the update()
method must throw an IllegalArgumentException
.
For the ADD
command, if the domain name exists, the IP address is updated and the previous IP address is returned. If no entry for the domain name was found, it is added and null
is returned.
For the DEL
command, if the domain name/IPAddress mapping is found, the entry is removed and the IP address is returned. If the domain name does not exist, the command is ignored and the method returns null
. If the domain name exists but the IPAddress does not match, a InputMismatchException
must be thrown.
Here is a sample update.txt file:
ADD 178.56.67.89 google.com ADD 178.56.67.112 google.com DEL 104.40.211.35 microsoft.com
To manually change an entry, the name of the domain and the corresponding IP address may be entered on the dialog box and click the Add or Delete button to add, update, or delete the entry.
The Simulator
Our DNS will be driven by a simple GUI simulator.
The main program should present a simple GUI as shown above. When it starts, it should perform the creation of a new DNS server object, but not actually start it. When the Start button is clicked, it starts the service as described above. If Stop is clicked, the service is stopped if the service was running and the dialog waits for another start.
If Update is clicked, the update (from update.txt) is performed as described above if and only if the service is running. If the service is running and Start is clicked, the action is ignored. If Exit is clicked, the simulation ends (the entire program stops running).
Undo/Redo
Based on your knowledge of stacks and queues, you may wish to add Undo and/or Redo buttons. These would allow the user to undo/redo Add and Delete commands. Ideally, the buttons would only be enabled when there is a command to be undone or redone.
Check with your instructor to see if this functionality is required or optional.
Benchmarking
You may wish to benchmark the performance when using a TreeMap
and compare it to the performance when using a HashMap
. You may need to make use of a larger dnsentries.txt file in order to see significant differences.
Check with your instructor to see if this functionality is required or optional.
Acknowledgements
This assignment was written by Prof. Jon Hopkins and Dr. Chris Taylor.
Lab Deliverables
See Prof. Hopkins for instructions Dr. Taylor's class: See below
See Prof. Ung for instructions