Lab
7
Start Now
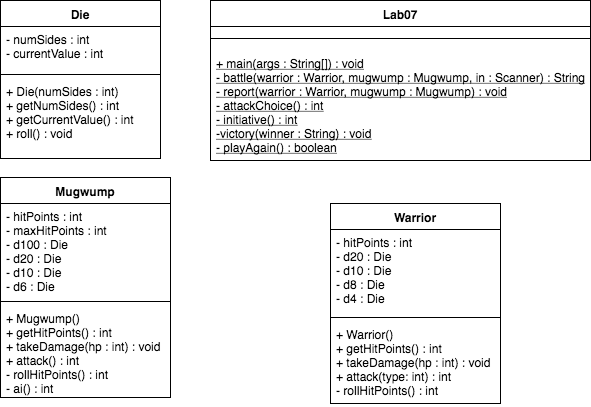
See your professor's instructions for details on
submission guidelines and due dates.
See Prof. Jones for instructions
See Dr. Retert for instructions
Dr. Taylor's class: submit using the form below
See Dr. Thomas for instructions
If you have any questions, consult your instructor.
## Acknowledgement
This laboratory assignment was developed by Prof. Sean Jones.
See Dr. Retert for instructions
Dr. Taylor's class: submit using the form below
See Dr. Thomas for instructions