Lab
8
Start Now
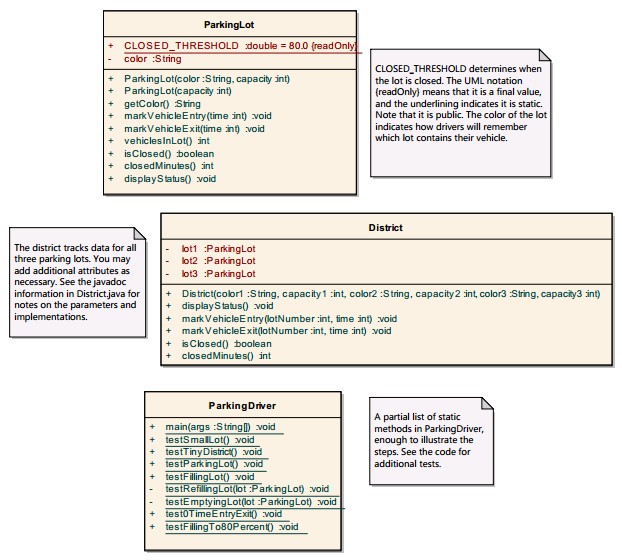
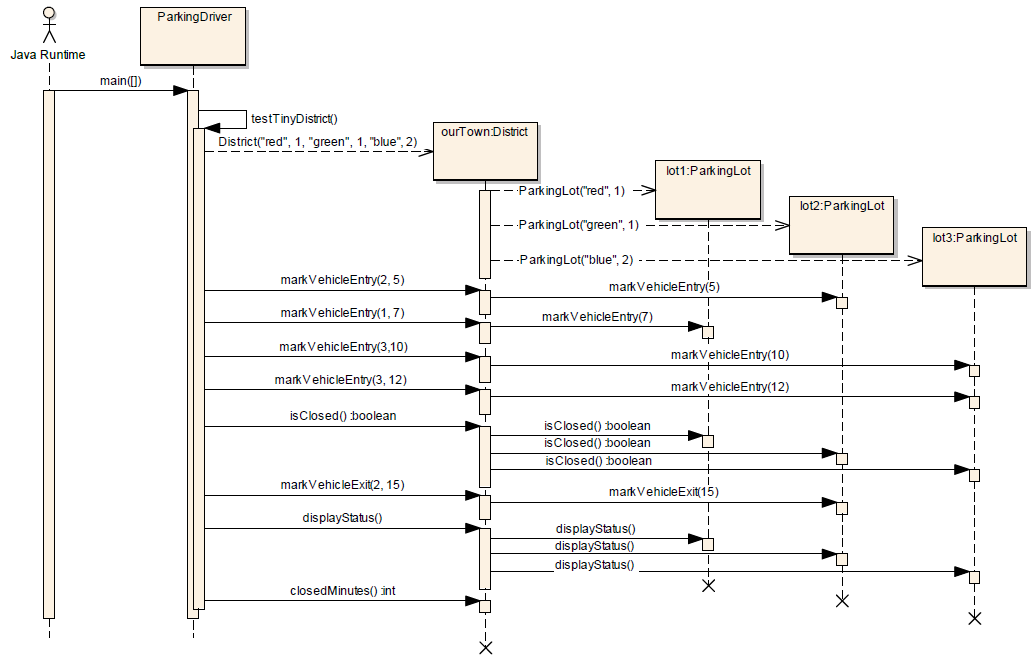
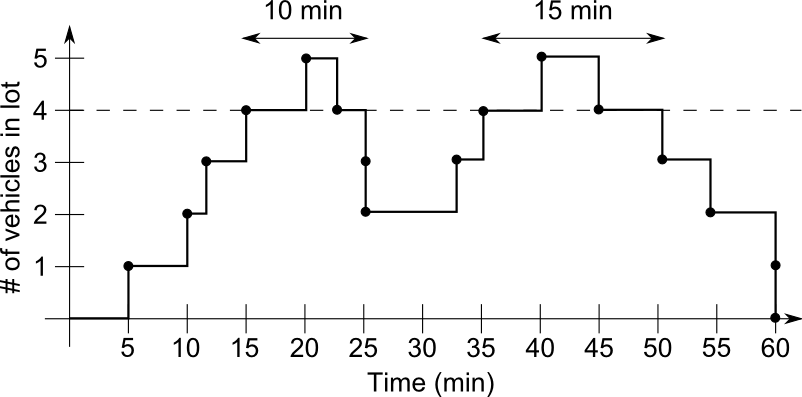
blacktop parking lot status: CLOSED Tiny district: red parking lot status: CLOSED green parking lot status: 0% blue parking lot status: CLOSED Lots were closed for 3 min. in tiny district. Testing ParkingLot test parking lot status: 75% test parking lot status: CLOSED test parking lot status: 50% test parking lot status: 0% Airport at time 7: brown parking lot status: 70% green parking lot status: CLOSED black parking lot status: 58.3% Airport at time 8: brown parking lot status: CLOSED green parking lot status: CLOSED black parking lot status: 58.3% Airport at time 10: brown parking lot status: CLOSED green parking lot status: CLOSED black parking lot status: CLOSED Testing heavier usage. At end of day, all lots closed 42 min. pink parking lot status: 68% blue parking lot status: CLOSED gray parking lot status: 20% All tests finished.## Lab Deliverables
See your professor's instructions for details on
submission guidelines and due dates.
See Prof. Jones for instructions
See Dr. Retert for instructions
Dr. Taylor's class: submit using the form below
See Dr. Thomas for instructions
If you have any questions, consult your instructor.
## Acknowledgement
This laboratory assignment was developed by
[Dr. Rob Hasker](https://faculty-web.msoe.edu/hasker/).
See Dr. Retert for instructions
Dr. Taylor's class: submit using the form below
See Dr. Thomas for instructions